mirror of
https://github.com/ryujinx-mirror/ryujinx.git
synced 2025-04-17 14:34:05 -05:00
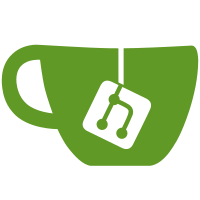
* Report base and extra sets from the backend * Pass texture set index everywhere * Key textures using set and binding (rather than just binding) * Start using extra sets for array textures * Shader cache version bump * Separate new commands, some PR feedback * Introduce new manual descriptor set reservation method that prevents it from being used by something else while owned by an array * Move bind extra sets logic to new method * Should only use separate array is MaximumExtraSets is not zero * Format whitespace
70 lines
2.0 KiB
C#
70 lines
2.0 KiB
C#
namespace Ryujinx.Graphics.Shader.IntermediateRepresentation
|
|
{
|
|
class TextureOperation : Operation
|
|
{
|
|
public const int DefaultCbufSlot = -1;
|
|
|
|
public SamplerType Type { get; set; }
|
|
public TextureFormat Format { get; set; }
|
|
public TextureFlags Flags { get; private set; }
|
|
|
|
public int Set { get; private set; }
|
|
public int Binding { get; private set; }
|
|
public int SamplerSet { get; private set; }
|
|
public int SamplerBinding { get; private set; }
|
|
|
|
public TextureOperation(
|
|
Instruction inst,
|
|
SamplerType type,
|
|
TextureFormat format,
|
|
TextureFlags flags,
|
|
int set,
|
|
int binding,
|
|
int compIndex,
|
|
Operand[] dests,
|
|
Operand[] sources) : base(inst, compIndex, dests, sources)
|
|
{
|
|
Type = type;
|
|
Format = format;
|
|
Flags = flags;
|
|
Set = set;
|
|
Binding = binding;
|
|
SamplerSet = -1;
|
|
SamplerBinding = -1;
|
|
}
|
|
|
|
public void TurnIntoArray(SetBindingPair setAndBinding)
|
|
{
|
|
Flags &= ~TextureFlags.Bindless;
|
|
Set = setAndBinding.SetIndex;
|
|
Binding = setAndBinding.Binding;
|
|
}
|
|
|
|
public void TurnIntoArray(SetBindingPair textureSetAndBinding, SetBindingPair samplerSetAndBinding)
|
|
{
|
|
TurnIntoArray(textureSetAndBinding);
|
|
|
|
SamplerSet = samplerSetAndBinding.SetIndex;
|
|
SamplerBinding = samplerSetAndBinding.Binding;
|
|
}
|
|
|
|
public void SetBinding(SetBindingPair setAndBinding)
|
|
{
|
|
if ((Flags & TextureFlags.Bindless) != 0)
|
|
{
|
|
Flags &= ~TextureFlags.Bindless;
|
|
|
|
RemoveSource(0);
|
|
}
|
|
|
|
Set = setAndBinding.SetIndex;
|
|
Binding = setAndBinding.Binding;
|
|
}
|
|
|
|
public void SetLodLevelFlag()
|
|
{
|
|
Flags |= TextureFlags.LodLevel;
|
|
}
|
|
}
|
|
}
|