mirror of
https://github.com/ryujinx-mirror/ryujinx.git
synced 2025-03-21 05:15:44 -05:00
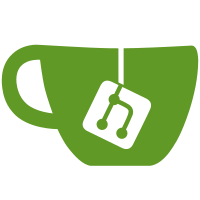
* misc: Move Ryujinx project to Ryujinx.Gtk3 This breaks release CI for now but that's fine. Signed-off-by: Mary Guillemard <mary@mary.zone> * misc: Move Ryujinx.Ava project to Ryujinx This breaks CI for now, but it's fine. Signed-off-by: Mary Guillemard <mary@mary.zone> * infra: Make Avalonia the default UI Should fix CI after the previous changes. GTK3 isn't build by the release job anymore, only by PR CI. This also ensure that the test-ava update package is still generated to allow update from the old testing channel. Signed-off-by: Mary Guillemard <mary@mary.zone> * Fix missing copy in create_app_bundle.sh Signed-off-by: Mary Guillemard <mary@mary.zone> * Fix syntax error Signed-off-by: Mary Guillemard <mary@mary.zone> --------- Signed-off-by: Mary Guillemard <mary@mary.zone>
71 lines
2.5 KiB
C#
71 lines
2.5 KiB
C#
using Avalonia;
|
|
using Avalonia.Controls;
|
|
using Avalonia.Controls.Notifications;
|
|
using Avalonia.Threading;
|
|
using Ryujinx.Ava.Common.Locale;
|
|
using Ryujinx.Common;
|
|
using System;
|
|
using System.Collections.Concurrent;
|
|
using System.Threading;
|
|
|
|
namespace Ryujinx.Ava.UI.Helpers
|
|
{
|
|
public static class NotificationHelper
|
|
{
|
|
private const int MaxNotifications = 4;
|
|
private const int NotificationDelayInMs = 5000;
|
|
|
|
private static WindowNotificationManager _notificationManager;
|
|
|
|
private static readonly BlockingCollection<Notification> _notifications = new();
|
|
|
|
public static void SetNotificationManager(Window host)
|
|
{
|
|
_notificationManager = new WindowNotificationManager(host)
|
|
{
|
|
Position = NotificationPosition.BottomRight,
|
|
MaxItems = MaxNotifications,
|
|
Margin = new Thickness(0, 0, 15, 40),
|
|
};
|
|
|
|
var maybeAsyncWorkQueue = new Lazy<AsyncWorkQueue<Notification>>(
|
|
() => new AsyncWorkQueue<Notification>(notification =>
|
|
{
|
|
Dispatcher.UIThread.Post(() =>
|
|
{
|
|
_notificationManager.Show(notification);
|
|
});
|
|
},
|
|
"UI.NotificationThread",
|
|
_notifications),
|
|
LazyThreadSafetyMode.ExecutionAndPublication);
|
|
|
|
_notificationManager.TemplateApplied += (sender, args) =>
|
|
{
|
|
// NOTE: Force creation of the AsyncWorkQueue.
|
|
_ = maybeAsyncWorkQueue.Value;
|
|
};
|
|
|
|
host.Closing += (sender, args) =>
|
|
{
|
|
if (maybeAsyncWorkQueue.IsValueCreated)
|
|
{
|
|
maybeAsyncWorkQueue.Value.Dispose();
|
|
}
|
|
};
|
|
}
|
|
|
|
public static void Show(string title, string text, NotificationType type, bool waitingExit = false, Action onClick = null, Action onClose = null)
|
|
{
|
|
var delay = waitingExit ? TimeSpan.FromMilliseconds(0) : TimeSpan.FromMilliseconds(NotificationDelayInMs);
|
|
|
|
_notifications.Add(new Notification(title, text, type, delay, onClick, onClose));
|
|
}
|
|
|
|
public static void ShowError(string message)
|
|
{
|
|
Show(LocaleManager.Instance[LocaleKeys.DialogErrorTitle], $"{LocaleManager.Instance[LocaleKeys.DialogErrorMessage]}\n\n{message}", NotificationType.Error);
|
|
}
|
|
}
|
|
}
|