mirror of
https://github.com/ryujinx-mirror/ryujinx.git
synced 2025-04-15 09:54:05 -05:00
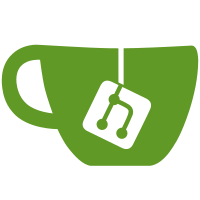
* Add area sampling scaler to allow for super-sampled anti-aliasing. * Area scaling filter doesn't have a scaling level. * Add further clarification to the tooltip on how to achieve supersampling. * ShaderHelper: Merge the two CompileProgram functions. * Convert tabs to spaces in area scaling shaders * Fixup Vulkan and OpenGL project files. * AreaScaling: Replace texture() by texelFetch() and use integer vectors. No functional difference, but it cleans up the code a bit. * AreaScaling: Delete unused sharpening level member. Also rename _scale to _sharpeningLevel for clarity and consistency. * AreaScaling: Delete unused scaleX/scaleY uniforms. * AreaScaling: Force the alpha to 1 when storing the pixel. * AreaScaling: Remove left-over sharpening buffer.
39 lines
1.2 KiB
C#
39 lines
1.2 KiB
C#
using OpenTK.Graphics.OpenGL;
|
|
using Ryujinx.Common.Logging;
|
|
|
|
namespace Ryujinx.Graphics.OpenGL.Effects
|
|
{
|
|
internal static class ShaderHelper
|
|
{
|
|
public static int CompileProgram(string shaderCode, ShaderType shaderType)
|
|
{
|
|
return CompileProgram(new string[] { shaderCode }, shaderType);
|
|
}
|
|
|
|
public static int CompileProgram(string[] shaders, ShaderType shaderType)
|
|
{
|
|
var shader = GL.CreateShader(shaderType);
|
|
GL.ShaderSource(shader, shaders.Length, shaders, (int[])null);
|
|
GL.CompileShader(shader);
|
|
|
|
GL.GetShader(shader, ShaderParameter.CompileStatus, out int isCompiled);
|
|
if (isCompiled == 0)
|
|
{
|
|
string log = GL.GetShaderInfoLog(shader);
|
|
Logger.Error?.Print(LogClass.Gpu, $"Failed to compile effect shader:\n\n{log}\n");
|
|
GL.DeleteShader(shader);
|
|
return 0;
|
|
}
|
|
|
|
var program = GL.CreateProgram();
|
|
GL.AttachShader(program, shader);
|
|
GL.LinkProgram(program);
|
|
|
|
GL.DetachShader(program, shader);
|
|
GL.DeleteShader(shader);
|
|
|
|
return program;
|
|
}
|
|
}
|
|
}
|